Update: WordPress Loop for a Custom Post Type
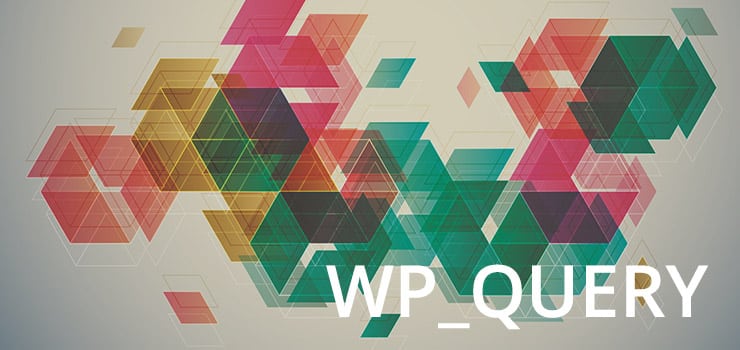
As our post on creating a loop for a custom post type was one of our most popular we thought we would add some updates to this and some better examples of how it works. You may find it better to start with this post if you haven’t read it already.
Our original post used an example of:
<?php $loop = new WP_Query( array( 'post_type' => 'yourposttypehere' // This is the name of your post type, 'posts_per_page' => 50 // This is the amount of posts per page you want to show ) ); while ( $loop->have_posts() ) : $loop->the_post(); ?> The stuff you want to loop goes in here for example: <div class="col-sm-4"> My column content </div> <?php endwhile; wp_reset_postdata(); ?>
Here the post type would be ‘yourposttypehere‘ which you would change to reflect your post type. For instance you could use ‘page‘ for pages, ‘post‘ for posts or anything else you have called your post type. If you are not sure what your post type is called then hover of the name of the post type in the admin and then look at the bottom of the screen in Chrome browser and you will see the post type name. i.e. http://yoursite.com/wp-admin/edit.php?post_type=yourposttypehere. If you are in a different browser then simply click on the post type name and when it opens up the page look in the URL bar for the correct post type name.
The parameter after post_type is something that we no longer recommend as this is setting the WP_Query to show every post in this post type on this page. You can see this by the setting of -1 and this could have a massive impact on your site if you have a lot of posts in the post type. Instead, we recommend setting a value that is high but that won’t bring back everything, something like 50 or 100 is most suitable.
You may also want to write the WP_Query so it is separated into different lines like the following example. This is purely your preference and won’t make any difference in how the query runs. Just make sure there is a comma after each parameter otherwise the query will break.
<?php $loop = new WP_Query( array( 'post_type' => 'yourposttypehere', 'posts_per_page' => 100 ) ); while ( $loop->have_posts() ) : $loop->the_post(); ?> The stuff you want to loop goes in here for example: <div class="col-sm-4"> My column content </div> <?php endwhile; wp_reset_postdata(); ?>
Other available parameters
There are lots of additional parameters you can add into the WP_Query to filter the query results even further. Here are a few quick examples:
Authors
‘author_name’ => ‘matt’
Filter the query by users with the name of ‘matt’
Category
‘category_name’ => ‘news’
Filter the query by post categories with the name of ‘news’
Taxonomy
‘your_taxonomy’ => ‘your_tax_term’
Filter the query by a taxonomy called ‘your_taxonomy’ with the term of ‘your_tax_term’
So an example of this might be:
<?php $loop = new WP_Query( array( 'post_type' => 'yourposttypehere', 'posts_per_page' => 5, 'category_name' => 'news', 'author_name' => 'matt' ) ); while ( $loop->have_posts() ) : $loop->the_post(); ?> The stuff you want to loop goes in here for example: <div class="col-sm-4"> My column content </div> <?php endwhile; wp_reset_postdata(); ?>
Here it is bringing back 5 posts from the ‘yourposttypehere’ post type in the category of ‘news’ which also have the author of ‘matt’.
Where to find the parameters to use…
If you are looking for where to find the parameters then your best reference is always the WordPress Codex and you can find the information about the WordPress WP_Query funcionality at https://codex.wordpress.org/Class_Reference/WP_Query.
Content within the loop
The loop within WordPress is its most basic and powerful function and WP_Query is an extension of this. Anything that you wrap within the query will get repeated. Here is an example:
<?php $loop = new WP_Query( array( 'post_type' => 'yourposttypehere', 'posts_per_page' => 100 ) ); while ( $loop->have_posts() ) : $loop->the_post(); ?> <div class="half-column"> <h2><?php the_title(); ?></h2> </div> <?php endwhile; wp_reset_postdata(); ?>
In this example, the div class of ‘half-column’ will get repeated for every time the loop brings back something. If there were 100 posts in the database in that post type then it would repeat 100 times and so on. Each column then shows the title of the post within the column
If the ‘half-column’ was then styled with a float: left and width of 50% then this will create a repeating 2 column layout. You will obviously have to do a bit more styling than this but hopefully, this will make sense. If you are using Bootstrap then a column class of col-sm-6 would be a similar effect.
Querying the database using WP_Query is so valuable and we use it on every site we build. It gives us the flexibility to create layouts and content with whatever characteristics we need.
How do you use WP_Query for querying post types?